
Here’s an example of using the limit() operation to limit the size of a stream of numbers: List numbers = Arrays.asList(1, 2, 3, 4, 5) numbers.stream(). It is often used to reduce the size of a large stream to a smaller one, which can make subsequent operations more efficient or easier to work with. The limit() operation is an intermediate operation in Java streams that limits the size of a stream to a maximum number of elements. We then use the sorted() method to sort the stream in alphabetical order, and finally we collect the sorted names into a new list using the collect() method. In this example, we first create a list of names, and then we obtain a stream from the list using the stream() method.
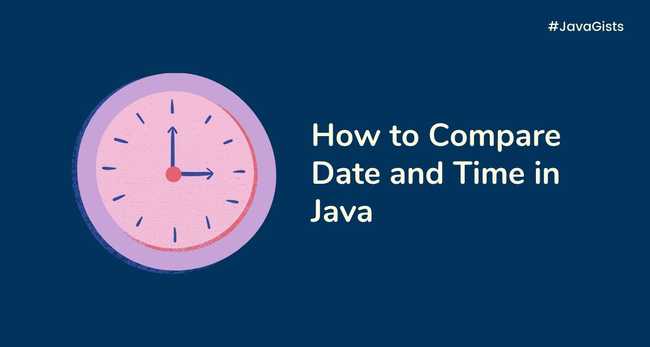
Here's an example of using sorted() to sort a list of names in alphabetical order: List names = Arrays.asList("John", "Mary", "David", "Samantha") List sortedNames = names.stream(). The sorted() method is an intermediate operation that returns a new stream with the elements sorted in natural order or a custom order specified by a comparator. We then use the distinct() method to filter the stream to only include unique numbers, and finally we collect the unique numbers into a new list using the collect() method. In this example, we first create a list of numbers, and then we obtain a stream from the list using the stream() method. Here's an example of using distinct() to get a list of unique numbers from a list: List numbers = Arrays.asList(1, 2, 3, 2, 4, 3, 5, 6, 5) List uniqueNumbers = numbers.stream(). The distinct() method is an intermediate operation that returns a new stream with the distinct elements in the stream. We then use the filter() method to filter the stream to only include even numbers, and finally we collect the filtered numbers into a new list using the collect() method. Here's an example of using filter() to filter a list of numbers to only include even numbers: List numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10) List evenNumbers = numbers.stream(). The filter() method is an intermediate operation that filters the stream based on a given predicate and returns a new stream with the filtered elements. We then use the map() method to transform each name to uppercase, and finally we collect the transformed names into a new list using the collect() method. Here's an example of using map() to convert a list of names to uppercase: List names = Arrays.asList("John", "Mary", "David", "Samantha") List uppercaseNames = names.stream(). The map() method is an intermediate operation that applies a function to each element in the stream and returns a new stream with the transformed elements. Intermediate Stream Methods and Examples map() Examples of terminal operations include forEach(), collect(), reduce(), and count(). Terminal operations are executed only when the stream pipeline is triggered, which happens when a terminal operation is called.

Terminal operations are operations that produce a result or side effect, such as printing or collecting the stream into a collection or a single value. Examples of intermediate operations include map(), filter(), distinct(), sorted(), and limit(). Intermediate operations return a new stream and do not modify the original stream. Intermediate operations are operations that transform, filter, or modify the stream in some way. There are two types of operations in Java Streams: intermediate and terminal. A stream can be obtained from a collection or an array using the stream() or parallelStream() methods, and once you have a stream, you can use a variety of methods to transform, filter, and aggregate the data. Streams are essentially a sequence of elements that can be processed in parallel or sequentially. Streams allow developers to express complex operations in a concise and readable way, and can lead to more efficient and parallelized code. Java Streams are a powerful and flexible way to process collections of data in a functional and declarative way.
